Personalized Conformal Prediction in Python: A Practical Guide
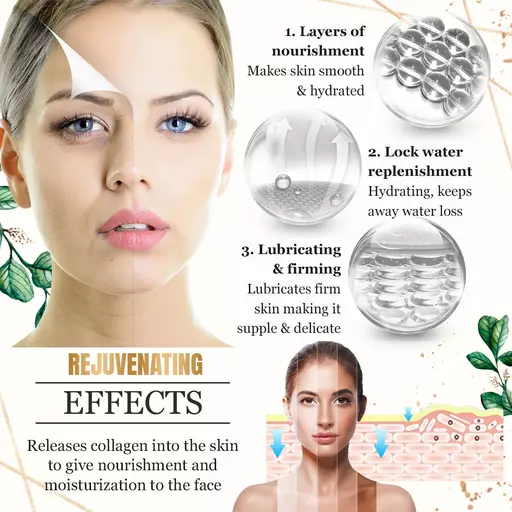
<!DOCTYPE html>
In the world of machine learning, making accurate predictions is crucial. However, understanding the uncertainty behind these predictions is equally important. This is where Conformal Prediction comes in, offering a powerful framework to quantify prediction uncertainty. This guide will walk you through implementing Personalized Conformal Prediction (PCP) in Python, a variant that tailors uncertainty estimates to individual data points, leading to more reliable and informative predictions. (Conformal Prediction, Uncertainty Quantification, Machine Learning)
What is Personalized Conformal Prediction?
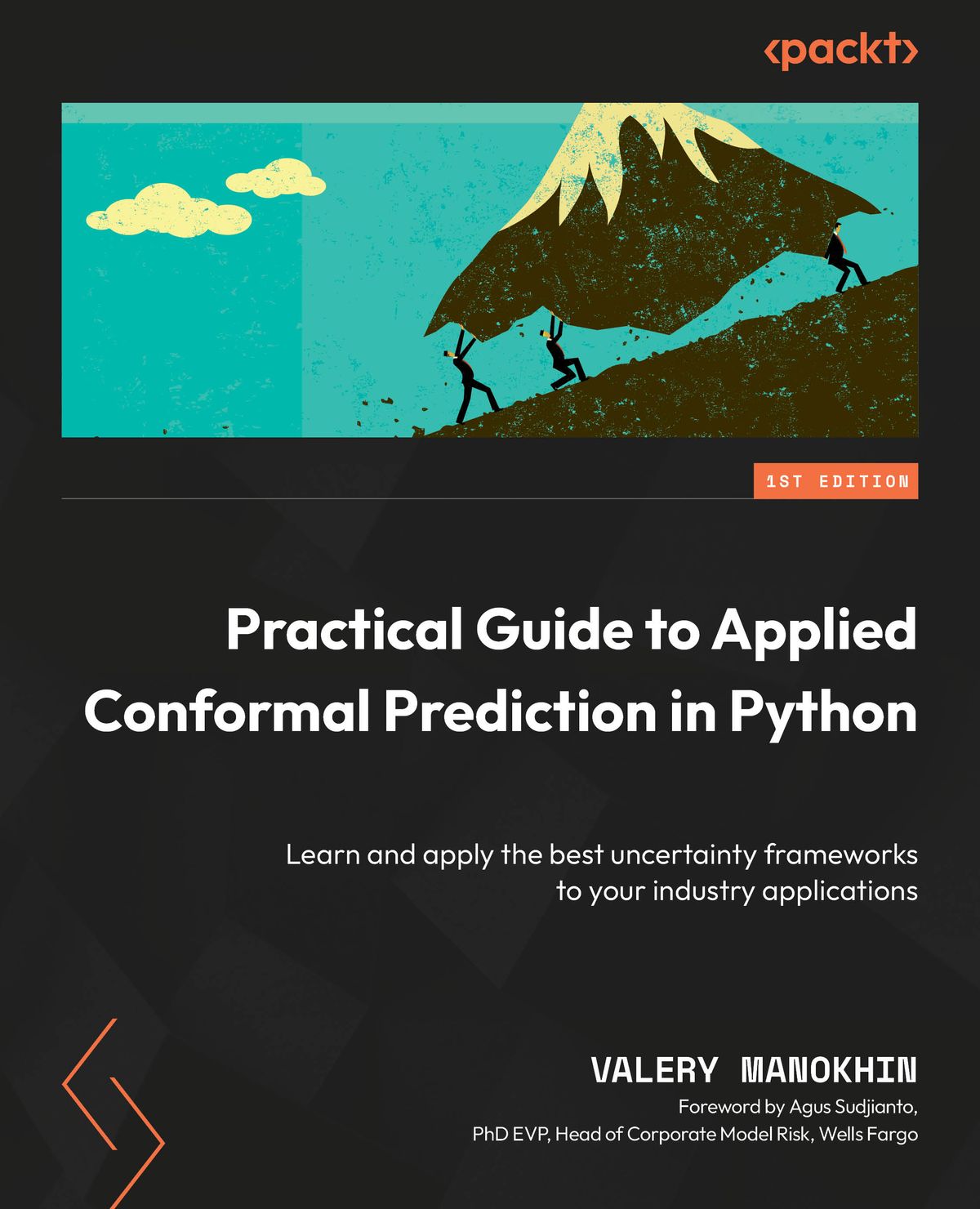
Traditional Conformal Prediction provides a single confidence interval for all predictions. Personalized Conformal Prediction (PCP) takes this a step further by adapting the confidence interval to the specific characteristics of each data point. This means that predictions for data points with high uncertainty will have wider intervals, while those with low uncertainty will have narrower ones. (Personalized Conformal Prediction, Confidence Intervals, Individualized Predictions)
Why Use Personalized Conformal Prediction?
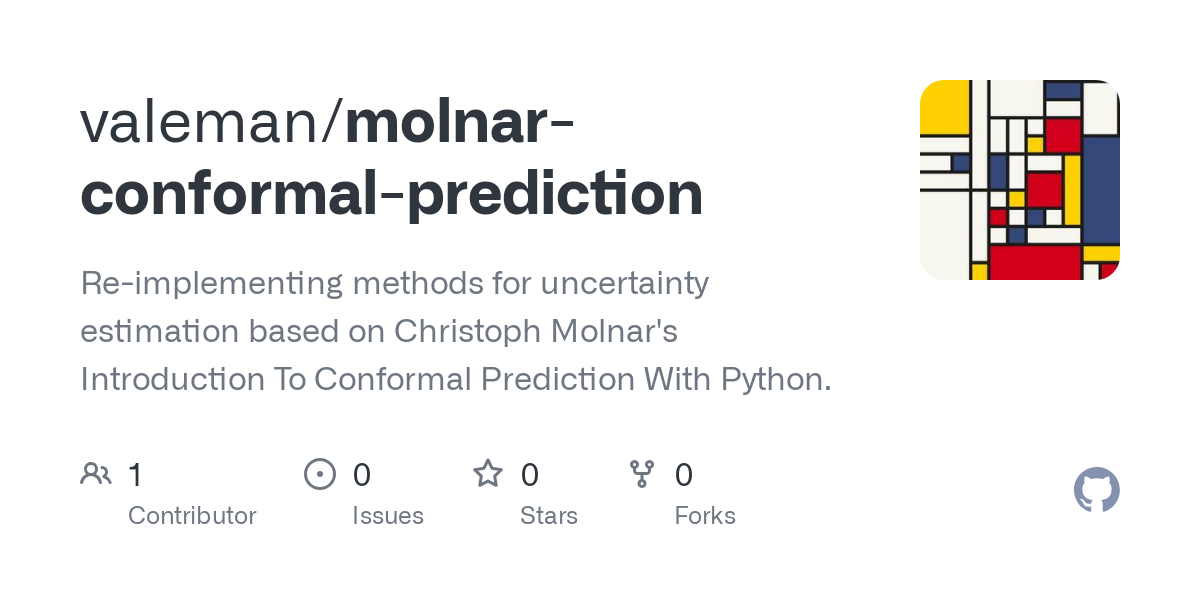
PCP offers several advantages over standard Conformal Prediction:
- Improved Accuracy: By tailoring uncertainty estimates, PCP can lead to more accurate predictions, especially for complex datasets.
- Enhanced Interpretability: Understanding the uncertainty associated with each prediction provides valuable insights into the model’s reliability.
- Better Decision-Making: PCP enables more informed decision-making by clearly communicating the confidence level of each prediction.
(Improved Accuracy, Enhanced Interpretability, Better Decision-Making)
Implementing PCP in Python
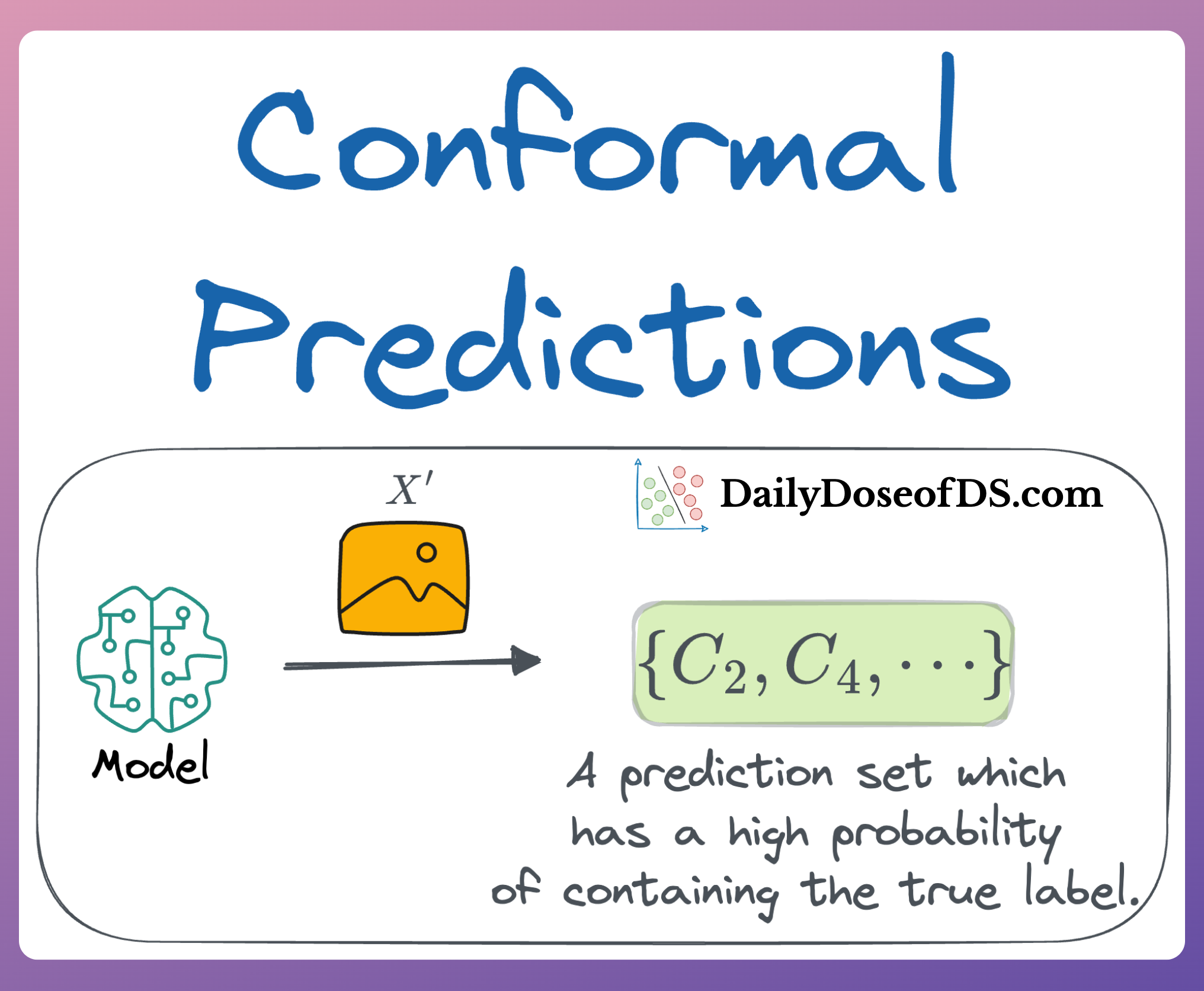
Let’s dive into the practical implementation of PCP using Python. We’ll use the popular conformal_prediction
library, which provides convenient tools for Conformal Prediction tasks.
1. Installation
First, install the necessary libraries:
pip install conformal_prediction scikit-learn
2. Data Preparation
Load your dataset and split it into training and calibration sets. The calibration set is crucial for determining the nonconformity scores used in PCP.
3. Model Training
Train your machine learning model on the training data. Any regression or classification model can be used with PCP.
4. Nonconformity Score Calculation
Calculate nonconformity scores for the calibration set. These scores measure how well the model’s predictions align with the actual target values.
5. Quantile Estimation
Determine the quantile of the nonconformity scores that corresponds to your desired confidence level (e.g., 90%). This quantile will be used to construct personalized prediction intervals.
6. Prediction with PCP
For new data points, calculate their nonconformity scores and compare them to the quantile obtained in step 5. The prediction interval for each data point is then determined based on this comparison.
📌 Note: The conformal_prediction
library provides functions to streamline these steps, making implementation more accessible.
Example Code Snippet
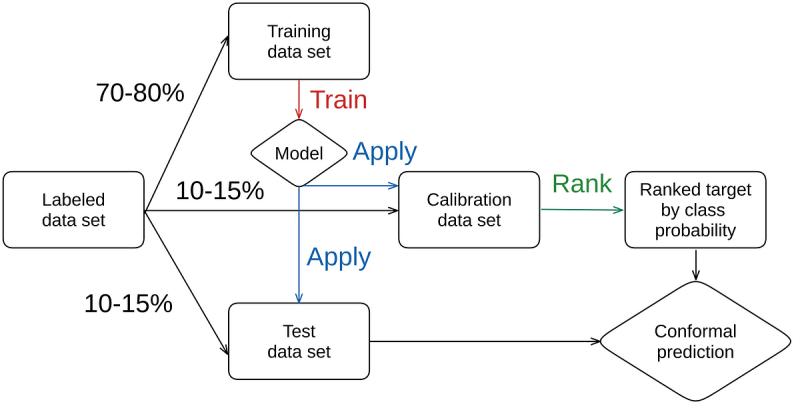
Here’s a simplified example using a linear regression model:
from conformal_prediction import ConformalRegressor
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
cp = ConformalRegressor(model, calibration_set=(X_cal, y_cal))
predictions, intervals = cp.predict(X_test, significance=0.1)
Key Considerations
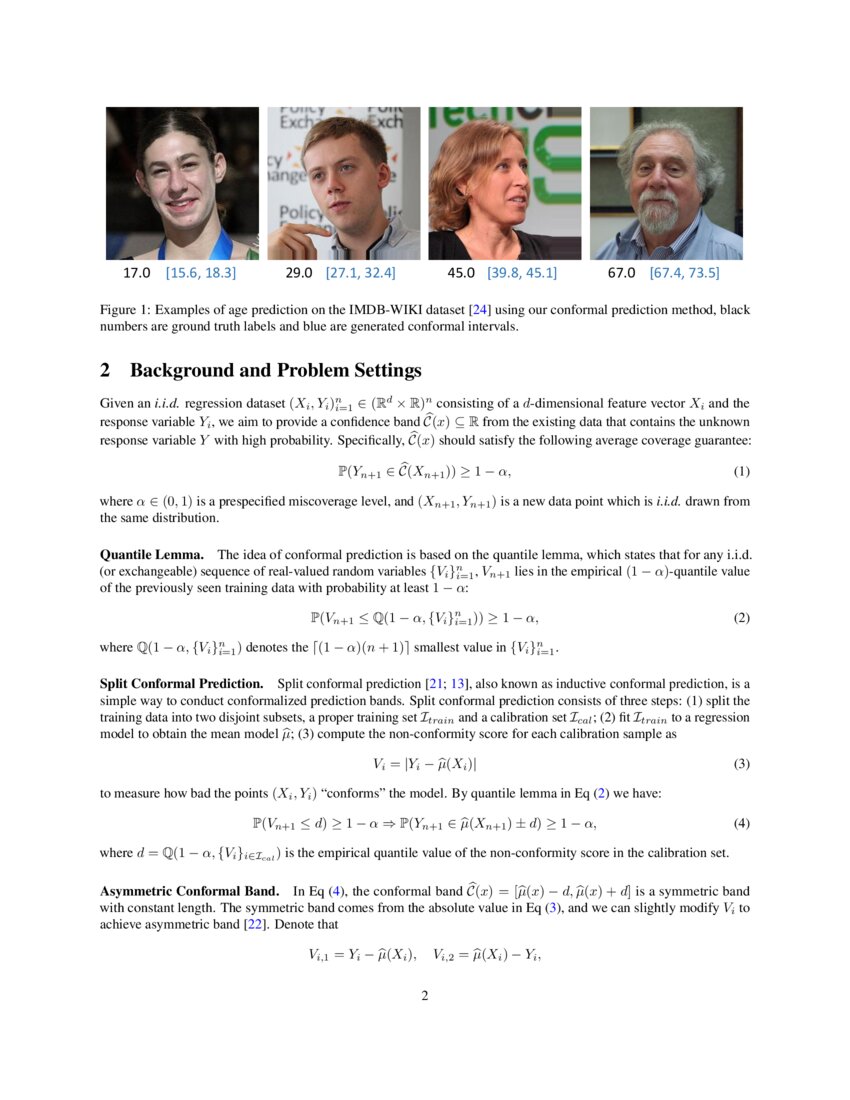
- Calibration Set Size: A larger calibration set generally leads to more accurate uncertainty estimates.
- Model Choice: The performance of PCP depends on the underlying machine learning model.
- Significance Level: Choose a significance level that balances confidence and interval width.
(Calibration Set Size, Model Choice, Significance Level)
By following these steps and considerations, you can effectively implement Personalized Conformal Prediction in Python, enhancing the reliability and interpretability of your machine learning models. (Personalized Conformal Prediction in Python, Reliable Predictions, Interpretable Machine Learning)
What are the main differences between Conformal Prediction and Personalized Conformal Prediction?
+Conformal Prediction provides a single confidence interval for all predictions, while Personalized Conformal Prediction tailors the interval to each individual data point based on its specific characteristics.
What types of machine learning models can be used with PCP?
+PCP can be used with any regression or classification model, as it focuses on quantifying uncertainty rather than the specific model architecture.
How does the size of the calibration set affect PCP performance?
+A larger calibration set generally leads to more accurate uncertainty estimates, as it provides a better representation of the data distribution.